woo.core
¶
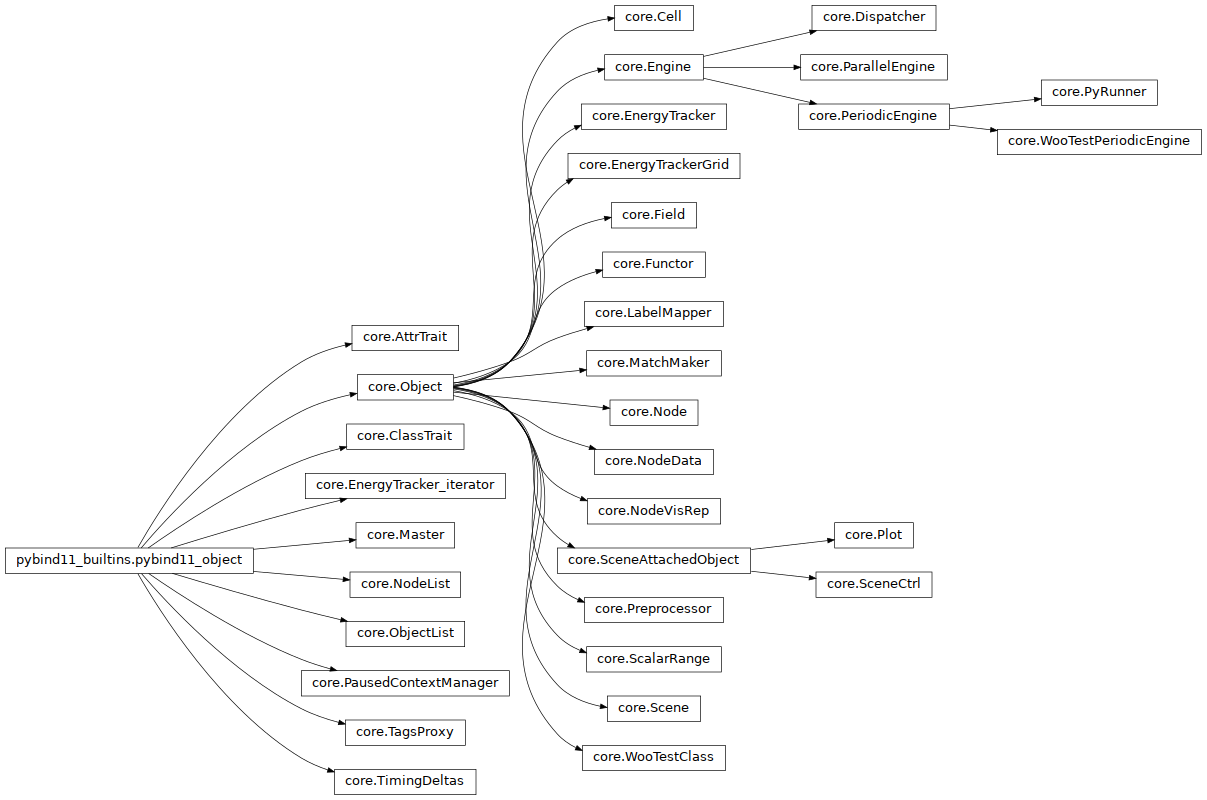
-
class
woo.core.
AttrTrait
¶ -
property
activeLabel
¶
-
property
altUnits
¶
-
property
bits
¶
-
property
choice
¶
-
property
className
¶
-
property
colormap
¶
-
property
cxxType
¶
-
property
deprecated
¶
-
property
dirname
¶
-
property
doc
¶
-
property
existingFilename
¶
-
property
filename
¶
-
property
hideIf
¶
-
property
ini
¶
-
property
multiUnit
¶
-
property
name
¶
-
property
namedEnum
¶
-
namedEnum_validValues
(self: woo.core.AttrTrait, pre0: str = '', post0: str = '', pre: str = '', post: str = '') → str¶ Valid values for named enum. pre and post are prefixed/suffixed to each possible value (used for formatting), pre0 and post0 are used with the first (primary/preferred) value.
-
property
noDump
¶
-
property
noGui
¶
-
property
noGuiResize
¶
-
property
noSave
¶
-
property
prefUnit
¶
-
property
pyByRef
¶
-
property
pyType
¶
-
property
range
¶
-
property
readonly
¶
-
property
rgbColor
¶
-
property
startGroup
¶
-
property
static
¶
-
property
triggerPostLoad
¶
-
property
unit
¶
-
property
-
class
woo.core.
ClassTrait
¶ -
property
doc
¶
-
property
docOther
¶
-
property
file
¶
-
property
intro
¶
-
property
line
¶
-
property
name
¶
-
property
title
¶
-
property
-
class
woo.core.
EnergyTracker_iterator
¶
-
class
woo.core.
Master
¶ -
property
api
¶ Current version of API (application programming interface) so that we can warn about possible incompatibilities, when comparing with
usesApi
. The number uses two decimal places for each part (major,minor,api), so e.g. 10177 is API 1.01.77. The correspondence with version number is loose.
-
property
cell
¶
-
checkApi
(self: woo.core.Master, minApi: int, msg: str, pyWarn: bool = True) → bool¶ Check whether the
currently used API
is at least minApi. If smaller, issue warning (which is either Python’sDeprecationWarning
or c++-level (log) warning depending on pyWarn) with link to the API changes page. Also issueFutureWarning
(or c++-level warning, depending on pyWarn) ifusesApi
is not set.
-
childClassesNonrecursive
(self: woo.core.Master, arg0: str) → list¶ Return list of all classes deriving from given class, as registered in the class factory
-
property
cmap
¶ Current colormap as (index,name) tuple; set by index or by name alone.
-
property
cmaps
¶ List available colormaps (by name)
-
property
compiledPyModules
¶
-
property
confDir
¶ Directory for storing various local configuration files (automatically set at startup)
-
static
deepcopy
(arg0: woo::Object, **kwargs) → woo::Object¶ Return a deep-copy of given object; this performs serialization+deserialization using temporary (RAM) storage; all objects are therefore created anew.
**kw
can be used to pass additional attributes which will be changed on the copy before it is returned; this allows one-liners likem2=m1.deepcopy(tanPhi=0)
.
-
disableGdb
(self: woo.core.Master) → None¶ Revert SEGV and ABRT handlers to system defaults.
-
property
dt
¶
-
property
energy
¶
-
property
engines
¶
-
exitNoBacktrace
(self: woo.core.Master, status: object = 0) → None¶ Disable SEGV handler and exit, optionally with given status number.
-
instance
= <woo.core.Master object>¶
-
isChildClassOf
(self: woo.core.Master, arg0: str, arg1: str) → bool¶ Tells whether the first class derives from the second one (both given as strings).
-
load
(*args, **kw)¶
-
loadTmp
(name='', quiet=None)¶ Load scene from temporary storage, assign to it
woo.master.scene
and return it.
-
loadTmpAny
(self: woo.core.Master, name: str = '') → woo::Object¶ Load any object from named temporary store.
-
lsTmp
(self: woo.core.Master) → list¶ Return list of all memory-saved simulations.
-
property
numThreads
¶ Maximum number of threads openMP can use.
-
pause
(*args, **kw)¶
-
property
periodic
¶
-
plugins
(self: woo.core.Master) → list¶ Return list of all plugins registered in the class factory.
-
property
realtime
¶ Return clock (human world) time the simulation has been running.
-
releaseScene
(self: woo.core.Master) → None¶ Release the scene object; only used internally at Python shutdown.
-
reload
(quiet=None, *args, **kw)¶ Reload master scene, using its
woo.core.Scene.lastSave
; assignswoo.master.scene
and returns the new scene object
-
reset
()¶
-
rmTmp
(self: woo.core.Master, name: str) → None¶ Remove memory-saved simulation.
-
run
(*args, **kw)¶
-
property
running
¶
-
save
(*args, **kw)¶
-
saveTmp
(name='', quiet=False)¶
-
saveTmpAny
(self: woo.core.Master, obj: woo::Object, name: str = '', quiet: bool = False) → None¶ Save any object to named temporary store; quiet will supress warning if the name is already used.
-
property
scene
¶
-
step
(*args, **kw)¶
-
property
timingEnabled
¶ Globally enable/disable timing services (see documentation of the
timing module
).
-
property
tmpFileDir
¶ Directory for temporary files; created automatically at startup.
-
tmpFilename
(self: woo.core.Master) → str¶ Return unique name of file in temporary directory which will be deleted when woo exits.
-
tmpToFile
(self: woo.core.Master, mark: str, fileName: str) → None¶ Save XML of
saveTmp
’d simulation into fileName.
-
tmpToString
(self: woo.core.Master, mark: str = '') → str¶ Return XML of
saveTmp
’d simulation as string.
-
property
trackEnergy
¶
-
property
usesApi
¶ API version this script is using; compared with
api
at some places to give helpful warnings. This variable can be set either from integer (e.g. 10177) or a Vector3i like(1,1,77)
.
-
property
usesApi_locations
¶
-
wait
(*args, **kw)¶
-
waitForScenes
(self: woo.core.Master) → None¶ Wait for master scene to finish, including the possibility of master scene being replaced by a different scene object. This is different from
Scene.wait
which will return when that particular scene object will have stopped. Internally, this method chains calls toScene.wait
as long aswoo.master.scene
is re-assigned (thus, everyScene
being de-assigned fromwoo.master.scene
must bestopped
, otherwise the call will never return.)
-
property
-
class
woo.core.
NodeList
(*args, **kwargs)¶ Overloaded function.
__init__(self: woo.core.NodeList) -> None
__init__(self: woo.core.NodeList, arg0: woo.core.NodeList) -> None
Copy constructor
__init__(self: woo.core.NodeList, arg0: iterable) -> None
-
append
(self: woo.core.NodeList, x: woo.core.Node) → None¶ Add an item to the end of the list
-
count
(self: woo.core.NodeList, x: woo.core.Node) → int¶ Return the number of times
x
appears in the list
-
extend
(*args, **kwargs)¶ Overloaded function.
extend(self: woo.core.NodeList, L: woo.core.NodeList) -> None
Extend the list by appending all the items in the given list
extend(self: woo.core.NodeList, L: iterable) -> None
Extend the list by appending all the items in the given list
-
insert
(self: woo.core.NodeList, i: int, x: woo.core.Node) → None¶ Insert an item at a given position.
-
pop
(*args, **kwargs)¶ Overloaded function.
pop(self: woo.core.NodeList) -> woo.core.Node
Remove and return the last item
pop(self: woo.core.NodeList, i: int) -> woo.core.Node
Remove and return the item at index
i
-
remove
(self: woo.core.NodeList, x: woo.core.Node) → None¶ Remove the first item from the list whose value is x. It is an error if there is no such item.
-
class
woo.core.
ObjectList
(*args, **kwargs)¶ Overloaded function.
__init__(self: woo.core.ObjectList) -> None
__init__(self: woo.core.ObjectList, arg0: woo.core.ObjectList) -> None
Copy constructor
__init__(self: woo.core.ObjectList, arg0: iterable) -> None
-
append
(self: woo.core.ObjectList, x: woo.core.Object) → None¶ Add an item to the end of the list
-
count
(self: woo.core.ObjectList, x: woo.core.Object) → int¶ Return the number of times
x
appears in the list
-
extend
(*args, **kwargs)¶ Overloaded function.
extend(self: woo.core.ObjectList, L: woo.core.ObjectList) -> None
Extend the list by appending all the items in the given list
extend(self: woo.core.ObjectList, L: iterable) -> None
Extend the list by appending all the items in the given list
-
insert
(self: woo.core.ObjectList, i: int, x: woo.core.Object) → None¶ Insert an item at a given position.
-
pop
(*args, **kwargs)¶ Overloaded function.
pop(self: woo.core.ObjectList) -> woo.core.Object
Remove and return the last item
pop(self: woo.core.ObjectList, i: int) -> woo.core.Object
Remove and return the item at index
i
-
remove
(self: woo.core.ObjectList, x: woo.core.Object) → None¶ Remove the first item from the list whose value is x. It is an error if there is no such item.
-
class
woo.core.
PausedContextManager
¶
-
class
woo.core.
TagsProxy
¶ -
has_key
(self: woo.core.TagsProxy, arg0: str) → bool¶
-
items
(self: woo.core.TagsProxy) → list¶
-
keys
(self: woo.core.TagsProxy) → list¶
-
update
(self: woo.core.TagsProxy, arg0: woo.core.TagsProxy) → None¶
-
values
(self: woo.core.TagsProxy) → list¶
-
-
class
woo.core.
TimingDeltas
¶ -
property
data
¶ Get timing data as list of tuples (label, execTime[nsec], execCount) (one tuple per checkpoint)
-
reset
(self: woo.core.TimingDeltas) → None¶ Reset timing information
-
property
Preprocessor¶
![digraph Preprocessor {
rankdir=LR;
margin=.2;
"Preprocessor" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Preprocessor"];
"woo.pre.horse.FallingHorse" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.pre.html#woo.pre.horse.Preprocessor"];
"Preprocessor" -> "woo.pre.horse.FallingHorse" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.pre.toys.NewtonsCradle" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.pre.html#woo.pre.toys.Preprocessor"];
"Preprocessor" -> "woo.pre.toys.NewtonsCradle" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.pre.ell2d.EllGroup" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.pre.html#woo.pre.ell2d.Preprocessor"];
"Preprocessor" -> "woo.pre.ell2d.EllGroup" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.pre.chute.DissipChute" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.pre.html#woo.pre.chute.Preprocessor"];
"Preprocessor" -> "woo.pre.chute.DissipChute" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.pre.psdrender.PsdRender" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.pre.html#woo.pre.psdrender.Preprocessor"];
"Preprocessor" -> "woo.pre.psdrender.PsdRender" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.pre.triax.TriaxTest" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.pre.html#woo.pre.triax.Preprocessor"];
"Preprocessor" -> "woo.pre.triax.TriaxTest" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.pre.cylTriax.CylTriaxTest" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.pre.html#woo.pre.cylTriax.Preprocessor"];
"Preprocessor" -> "woo.pre.cylTriax.CylTriaxTest" [arrowsize=0.5,style="setlinewidth(0.5)"]
}](_images/graphviz-1bb35fd9a0d0e2cbbf084d2753e429b18890f4c0.png)
-
class
woo.core.
Preprocessor
(*args, **kwargs)¶ Subclasses of this class generate a Scene object when called, based on their attributes.
Overloaded function.
__init__(self: woo.core.Preprocessor) -> None
__init__(self: woo.core.Preprocessor, *args, **kwargs) -> None
-
title
(= '')¶ Simulation title, will be assigned to the {title} tag, unless running in batch (where the batch title will be used.
[type: string]
-
__call__
(self: woo.core.Preprocessor) → woo.core.Scene¶
ScalarRange¶
-
class
woo.core.
ScalarRange
(*args, **kwargs)¶ Store and share range of scalar values
Overloaded function.
__init__(self: woo.core.ScalarRange) -> None
__init__(self: woo.core.ScalarRange, *args, **kwargs) -> None
-
mnmx
(= Vector2(inf, -inf))¶ Packed minimum and maximum values; adjusting from python sets
autoAdjust
to false automatically.[type: Vector2r]
-
logMnmx
(= Vector2(1.2300495001352267e242, 6.3259690378579e-310))¶ Logs of mnmx values, to avoid computing logarithms all the time; computed via cacheLogs.
[type: Vector2r, not saved, not accessible from python]
-
flags
(= 8)¶ Flags for this range.
[type: int, bit accessors: log, reversed, symmetric, autoAdjust, hidden, clip, used]
-
dispPos
(= Vector2i(-1000, -1000))¶ Where is this range displayed on the OpenGL canvas; initially out of range, will be reset automatically.
[type: Vector2i, not shown in the UI]
-
length
(= 200.0)¶ Length on the display; if negative, it is fractional relative to view width/height
[type: Real, not shown in the UI]
-
landscape
(= False)¶ Make the range display with landscape orientation
[type: bool, not shown in the UI]
-
label
(= '')¶ Short name of this range.
[type: std::string]
-
cmap
(= -1)¶ Colormap to be used.
[type: int, named enum, possible values are: ‘default’ (‘’; -1), ‘coolwarm’ (0), ‘3gauss’ (1), ‘3saw’ (2), ‘banded’ (3), ‘blue_red’ (4), ‘blu_red’ (5), ‘bright’ (6), ‘bw’ (7), ‘default’ (8), ‘detail’ (9), ‘extrema’ (10), ‘helix2’ (11), ‘helix’ (12), ‘hotres’ (13), ‘jaisn2’ (14), ‘jaisnb’ (15), ‘jaisnc’ (16), ‘jaisnd’ (17), ‘jaison’ (18), ‘jet’ (19), ‘manga’ (20), ‘rainbow’ (21), ‘roullet’ (22), ‘ssec’ (23), ‘wheel’ (24), ‘red-green-blue’ (25), ‘viridis’ (26)]
-
norm
(self: woo.core.ScalarRange, val: float, clamp: bool = True) → float¶ Return value of the argument normalized to 0..1 range; the value is not clamped to 0..1 however: if autoAdjust is false, it can fall outside.
-
reset
(self: woo.core.ScalarRange) → None¶
EnergyTrackerGrid¶
-
class
woo.core.
EnergyTrackerGrid
(*args, **kwargs)¶ Storage of spatially located energy values.
Overloaded function.
__init__(self: woo.core.EnergyTrackerGrid) -> None
__init__(self: woo.core.EnergyTrackerGrid, *args, **kwargs) -> None
-
box
(= AlignedBox3((1.7976931348623157e308, 1.7976931348623157e308, 1.7976931348623157e308), (-1.7976931348623157e308, -1.7976931348623157e308, -1.7976931348623157e308)))¶ Part of space which we monitor.
[type: AlignedBox3r, read-only in python]
-
cellSize
(= nan)¶ Size of one cell in the box (in all directions); will be satisfied exactly, at the expense of slightly growing
box
. Do not change.[type: Real, read-only in python]
-
boxCells
(= Vector3i(0, 0, 0))¶ Number of cells in the box (computed automatically).
[type: Vector3i, read-only in python]
-
data
¶ Grid data – 5d since each 3d point contains multiple energies, and there are multiple threads writing concurrently.
[type: boost_multi_array_real_5, not accessible from python]
-
vtkExport
(self: woo.core.EnergyTrackerGrid, out: str, names: List[str]) → None¶ Export data into VTK grid file out, using names to name the arrays exported.
Scene¶
TODO
Scene¶
-
class
woo.core.
Scene
(*args, **kwargs)¶ Object comprising the whole simulation.
Overloaded function.
-
dt
(= nan)¶ Current timestep for integration.
[type: Real, unit: s]
-
nextDt
(= nan)¶ Timestep for the next step (if not NaN,
dt
is automatically replaced by this value at the end of the step).[type: Real, unit: s]
-
dtSafety
(= 0.9)¶ Safety factor for automatically-computed timestep.
[type: Real]
-
throttle
(= 0.0)¶ Insert this delay before each step when running in loop; useful for slowing down simulations for visual inspection if they happen too fast.
Note
Negative values will not make the simulation run faster.
[type: Real]
-
step
(= 0)¶ Current step number
[type: long, read-only in python]
-
subStepping
(= False)¶ Whether we currently advance by one engine in every step (rather than by single run through all engines).
[type: bool]
-
subStep
(= -1)¶ Number of sub-step; not to be changed directly. -1 means to run loop prologue (cell integration), 0…n-1 runs respective engines (n is number of engines), n runs epilogue (increment step number and time.
[type: int, read-only in python]
-
time
(= 0.0)¶ Simulation time (virtual time) [s]
[type: Real, unit: s, read-only in python]
-
stopAtStep
(= 0)¶ Iteration after which to stop the simulation.
[type: long]
-
stopAtTime
(= nan)¶ time
around which to stop the simulation.Note
This value is not exact, has the granularity of \(\Dt\): simulation will stopped at the moment when
stopAtTime
≤time
<dt
+stopAtTime
. This condition may have some corner cases due to floating-point comparisons involved.[type: Real]
-
stopAtHook
(= '')¶ Python command given as string executed when
stopAtTime
orstopAtStep
cause the simulation to be stopped.Note
This hook will not be run when a running simulation is paused manually, or through S.pause in the script. To trigger this hook from within a
PyRunner
, do something likeS.stopAtStep=S.step
which will activate it when the current step finishes.[type: string]
-
isPeriodic
(= False)¶ Whether periodic boundary conditions are active.
[type: bool, not accessible from python]
-
trackEnergy
(= False)¶ Whether energies are being tracked.
[type: bool]
-
deterministic
(= False)¶ Hint for engines to order (possibly at the expense of performance) arithmetic operations to be independent of thread scheduling; this results in simulation with the same initial conditions being always the same. This is disabled by default, because of performance issues. Note that deterministic result is not “more correct” (neither physically, nor theoretically) than other result with different operation ordering; it is only self-consistent and feels better.
[type: bool]
-
selfTestEvery
(= 0)¶ Periodicity with which consistency self-tests will be run; 0 to run only in the very first step, negative to disable.
[type: int]
-
clDev
(= Vector2i(-1, -1))¶ OpenCL device to be used; if (-1,-1) (default), no OpenCL device will be initialized until requested. Saved simulations should thus always use the same device when re-loaded.
[type: Vector2i]
-
_clDev
(= Vector2i(-1, -1))¶ OpenCL device which is really initialized (to detect whether clDev was changed manually to avoid spurious re-initializations from postLoad
[type: Vector2i, not saved, read-only in python]
-
boxHint
(= AlignedBox3((1.7976931348623157e308, 1.7976931348623157e308, 1.7976931348623157e308), (-1.7976931348623157e308, -1.7976931348623157e308, -1.7976931348623157e308)))¶ Hint for displaying the scene; overrides node-based detection. Set an element to empty box to disable.
[type: AlignedBox3r]
-
runInternalConsistencyChecks
(= True)¶ Run internal consistency check, right before the very first simulation step.
[type: bool, not accessible from python]
Arbitrary key=value associations (tags like mp3 tags: author, date, version, description etc.)
[type: StrStrMap, not accessible from python]
-
labels
(= <LabelMapper @ 0x15bbf90>)¶ Atrbitrary key=object, key=list of objects, key=py::object associations which survive saving/loading. Labeled objects are automatically added to this container. This object is more conveniently accessed through the
lab
attribute, which exposes the mapping as attributes of that object rather than as a dictionary.[type: shared_ptr<
LabelMapper
>, not shown in the UI, read-only in python]
-
uiBuild
(= '')¶ Command to run when a new main-panel UI should be built for this scene (called when the Controller is opened with this simulation, or the simulation is new to the controller).
[type: string]
-
engines
(= [])¶ Engines sequence in the simulation (direct access to the c++ sequence is shadowed by python property which access it indirectly).
[type: vector<shared_ptr<Engine>>]
-
_nextEngines
(= [])¶ Engines to be used from the next step on; is returned transparently by S.engines if in the middle of the loop (controlled by subStep>=0).
[type: vector<shared_ptr<Engine>>, not accessible from python]
-
energy
(= <EnergyTracker @ 0x14d4500>)¶ Energy values, if energy tracking is enabled.
[type: shared_ptr<
EnergyTracker
>, not shown in the UI, read-only in python]
-
fields
(= [])¶ Defined simulation fields.
[type: vector<shared_ptr<Field>>, not shown in the UI]
-
cell
(= <Cell @ 0x15c8c30>)¶ Information on periodicity; only should be used if Scene::isPeriodic.
[type: shared_ptr<
Cell
>, not accessible from python]
-
lastSave
(= '')¶ Name under which the simulation was saved for the last time; used for reloading the simulation. Updated automatically, don’t change.
[type: std::string, read-only in python]
-
preSaveDuration
(= 0)¶ Wall clock duration this Scene was alive before being saved last time; this count is incremented every time the scene is saved. When Scene is loaded, it is used to construct clock0 as current_local_time - lastSecDuration.
[type: long, not shown in the UI, read-only in python]
-
dispParams
(= [])¶ Saved display states.
[type: vector<shared_ptr<DisplayParameters>>, not shown in the UI]
-
gl
(= None)¶ Settings related to rendering; default instance is created on-the-fly when requested from Python.
[type: shared_ptr<
GlSetup
>, not accessible from python]
-
glDirty
(= True)¶ Flag to re-initalize functors, colorscales and restore QGLViewer before rendering.
[type: bool, read-only in python]
-
ranges
(= [])¶ User-defined
ScalarRange
objects, to be rendered as colormaps.[type: vector<shared_ptr<ScalarRange>>]
-
autoRanges
(= [])¶ ScalarRange
colormaps automatically obtained from renderes and engines; displayed only when actually used.[type: vector<shared_ptr<ScalarRange>>, read-only in python]
-
any
(= ObjectList[])¶ Storage for arbitrary Objects; meant for storing and loading static objects like Gl1_* functors to restore their parameters when scene is loaded.
[type: vector<shared_ptr<Object>>]
-
pre
¶ Preprocessor used for generating this simulation; to be only used in user scripts to query preprocessing parameters, not in c++ code.
[type: py::object, not shown in the UI]
-
plot
(= <Plot @ 0x15c76b0>)¶ Data and settings for plots.
[type: shared_ptr<
Plot
>, not shown in the UI]
-
property
duration
¶ Number of (wall clock) seconds this instance is alive (including time before being loaded from file
-
ensureCl
(self: woo.core.Scene) → None¶ [for debugging] Initialize the OpenCL subsystem (this is done by engines using OpenCL, but trying to do so in advance might catch errors earlier)
-
expandTags
(self: woo.core.Scene, arg0: str) → str¶ Expand
tags
written as{tagName}
, returns the expanded string.
-
getRange
(self: woo.core.Scene, arg0: str) → woo.core.ScalarRange¶ Retrieve a ScalarRange object by its label
-
one
(self: woo.core.Scene) → None¶
-
paused
(self: woo.core.Scene, allowBg: bool = False) → Scene::PausedContextManager¶ Return paused context manager; when allowBg is True, the context manager is a no-op in the engine background thread and works normally when called from other threads).
-
property
periodic
¶ Set whether the scene is periodic or not
-
saveTmp
(self: woo.core.Scene, slot: str = '', quiet: bool = False) → None¶ Save into a temporary slot inside Master (loadable with O.loadTmp)
-
selfTest
(self: woo.core.Scene) → None¶ Run self-tests (they are usually run automatically with, see
selfTestEvery
).
-
setLastSave
(self: woo.core.Scene, arg0: str) → None¶
-
stop
(self: woo.core.Scene) → None¶
-
wait
(self: woo.core.Scene) → None¶
-
SceneAttachedObject¶
![digraph SceneAttachedObject {
rankdir=LR;
margin=.2;
"SceneAttachedObject" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.SceneAttachedObject"];
"Plot" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.SceneAttachedObject"];
"SceneAttachedObject" -> "Plot" [arrowsize=0.5,style="setlinewidth(0.5)"] "SceneCtrl" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.SceneAttachedObject"];
"SceneAttachedObject" -> "SceneCtrl" [arrowsize=0.5,style="setlinewidth(0.5)"]
}](_images/graphviz-6381ff7a03e6b61ac7bf938e238d8eef0f91bd8a.png)
-
class
woo.core.
SceneAttachedObject
(*args, **kwargs)¶ Parent class for object uniquely attached to one scene, for convenience of derived classes.
Overloaded function.
Plot¶
Object
→ SceneAttachedObject
→ Plot
-
class
woo.core.
Plot
(*args, **kwargs)¶ Storage for plots updated during simulation.
Overloaded function.
-
data
(= {})¶ Global dictionary containing all data values, common for all plots, in the form {‘name’:[value,…],…}. Data should be added using plot.addData function. All [value,…] columns have the same length, they are padded with NaN if unspecified.
[type: py::dict]
-
imgData
(= {})¶ Dictionary containing lists of strings, which have the meaning of images corresponding to respective
woo.plot.data
rows. Seewoo.plot.plots
on how to plot images.[type: py::dict]
-
plots
(= {})¶ dictionary x-name -> (yspec,…), where yspec is either y-name or (y-name,’line-specification’). If
(yspec,...)
isNone
, then the plot has meaning of image, which will be taken from respective field ofwoo.plot.imgData
.[type: py::dict]
-
labels
(= {})¶ Dictionary converting names in data to human-readable names (TeX names, for instance); if a variable is not specified, it is left untranslated.
[type: py::dict]
-
xylabels
(= {})¶ Dictionary of 2-tuples specifying (xlabel,ylabel) for respective plots; if either of them is None, the default auto-generated title is used.
[type: py::dict]
-
legendLoc
(= ('upper left', 'upper right'))¶ Location of the y1 and y2 legends on the plot, if y2 is active.
[type: py::tuple]
-
axesWd
(= 1.0)¶ Linewidth (in points) to make x and y axes better visible; not activated if non-positive.
[type: Real]
-
currLineRefs
(= [])¶ References to axes which are being shown. Internal use only.
[type: py::list, not shown in the UI, not saved]
-
annotateFmt
(= ' {xy[1]:.4g}')¶ Format for annotations in plots; if empty, no annotation is shown; has no impact on existing plots. xy is 2-tuple of the current point in data space.
[type: string]
-
addData
(*d_in, **kw)¶ Add data from arguments name1=value1,name2=value2 to woo.plot.data. (the old {‘name1’:value1,’name2’:value2} is deprecated, but still supported)
New data will be padded with nan’s, unspecified data will be nan (nan’s don’t appear in graphs). This way, equal length of all data is assured so that they can be plotted one against any other.
>>> S=woo.master.scene >>> from pprint import pprint >>> S.plot.resetData() >>> S.plot.addData(a=1) >>> S.plot.addData(b=2) >>> S.plot.addData(a=3,b=4) >>> pprint(S.plot.data) {'a': [1, nan, 3], 'b': [nan, 2, 4]}
Some sequence types can be given to addData; they will be saved in synthesized columns for individual components.
>>> S.plot.resetData() >>> S.plot.addData(c=Vector3(5,6,7),d=Matrix3(8,9,10, 11,12,13, 14,15,16)) >>> pprint(S.plot.data) {'c_norm': [10.488...], 'c_x': [5.0], 'c_y': [6.0], 'c_z': [7.0], 'd_xx': [8.0], 'd_xy': [9.0], 'd_xz': [10.0], 'd_yx': [11.0], 'd_yy': [12.0], 'd_yz': [13.0], 'd_zx': [14.0], 'd_zy': [15.0], 'd_zz': [16.0]}
-
autoData
(**kw)¶ Add data by evaluating contents of
woo.core.Plot.plots
. Expressions rasing exceptions will be handled gracefully, but warning is printed for each.>>> from woo import plot; from woo.dem import *; from woo.core import * >>> from pprint import pprint >>> S=Scene(fields=[DemField(gravity=(0,0,-10))]) >>> S.plot.plots={'S.step':('S.time',None,'numParticles=len(S.dem.par)')} >>> S.plot.autoData() >>> pprint(S.plot.data) {'S.step': [0], 'S.time': [0.0], 'numParticles': [0]}
Note that each item in
woo.core.Plot.plots
can bean expression to be evaluated (using the
eval
builtin);name=expression
string, wherename
will appear as label in plots, and expression will be evaluated each time;a dictionary-like object – current keys are labels of plots and current values are added to
woo.core.Plot.data
. The contents of the dictionary can change over time, in which case new lines will be created as necessary.
A simple simulation with plot can be written in the following way; note how the energy plot is specified.
>>> from woo import plot, utils >>> S=Scene(fields=[DemField(gravity=(0,0,-10))]) >>> S.plot.plots={'i=S.step':('**S.energy','total energy=S.energy.total()',None,'rel. error=S.energy.relErr()')} >>> # we create a simple simulation with one ball falling down >>> S.dem.par.add(Sphere.make((0,0,0),1,mat=utils.defaultMaterial())) 0 >>> S.engines=[Leapfrog(damping=.4,reset=True), ... # get data required by plots at every step ... PyRunner(1,'S.plot.autoData()') ... ] >>> S.trackEnergy=True >>> S.run(3,True) >>> pprint(S.plot.data) {'grav': [0.0, 0.0, -20.357...], 'i': [0, 1, 2], 'kinetic': [0.0, 1.526..., 13.741...], 'nonviscDamp': [nan, nan, 8.143...], 'rel. error': [0.0, 1.0, 0.0361...], 'total energy': [0.0, 1.526..., 1.526...]}
(Source code, png, hires.png, pdf)
-
plot
(noShow=False, subPlots=True)¶ Do the actual plot, which is either shown on screen (and nothing is returned: if noShow is
False
) or, if noShow isTrue
, returned list of matplotlib’s Figure objects.You can use
>>> import woo,woo.core,os >>> S=woo.core.Scene() >>> S.plot.plots={'foo':('bar',)} >>> S.plot.addData(foo=1,bar=2) >>> somePdf=woo.master.tmpFilename()+'.pdf' >>> S.plot.plot(noShow=True)[0].savefig(somePdf) >>> os.path.exists(somePdf) True
to save the figure to file automatically.
-
reset
()¶ Reset all plot-related variables (data, plots, labels)
-
resetData
()¶ Reset all plot data; keep plots and labels intact.
-
reverseData
()¶ Reverse woo.core.Plot.data order.
Useful for tension-compression test, where the initial (zero) state is loaded and, to make data continuous, last part must end in the zero state.
-
saveDataTxt
(fileName, vars=None)¶ Save plot data into a (optionally compressed) text file. The first line contains a comment (starting with
#
) giving variable name for each of the columns. This format is suitable for being loaded for further processing (outside woo) withnumpy.genfromtxt
function, which recognizes those variable names (creating numpy array with named entries) and handles decompression transparently.>>> import woo, woo.core >>> from pprint import pprint >>> S=woo.core.Scene() >>> S.plot.addData(a=1,b=11,c=21,d=31) # add some data here >>> S.plot.addData(a=2,b=12,c=22,d=32) >>> pprint(S.plot.data) {'a': [1, 2], 'b': [11, 12], 'c': [21, 22], 'd': [31, 32]} >>> txt=woo.master.tmpFilename()+'.txt.gz' >>> S.plot.saveDataTxt(txt,vars=('a','b','c')) >>> import numpy >>> d=numpy.genfromtxt(txt,dtype=None,names=True) >>> d['a'] array([1, 2]) >>> d['b'] array([11, 12])
- Parameters
fileName – file to save data to; if it ends with
.bz2
/.gz
, the file will be compressed using bzip2 / gzip.vars – Sequence (tuple/list/set) of variable names to be saved. If
None
(default), all variables inwoo.core.Plot
are saved.
-
saveGnuplot
(baseName, term='wxt', extension=None, timestamp=False, comment=None, title=None, varData=False, timeStamp=True)¶ Save data added with
woo.plot.addData
into (compressed) file and create .gnuplot file that attempts to mimick plots specified withwoo.plot.plots
.- Parameters
baseName – used for creating baseName.gnuplot (command file for gnuplot), associated
baseName.data.bz2
(data) and output files (if applicable) in the formbaseName.[plot number].extension
term – specify the gnuplot terminal; defaults to
x11
, in which case gnuplot will draw persistent windows to screen and terminate; other useful terminals arepng
,cairopdf
and so onextension – extension for
baseName
defaults to terminal name; fine for png for example; if you usecairopdf
, you should also sayextension='pdf'
howevertimestamp (bool) – append numeric time to the basename
varData (bool) – whether file to plot will be declared as variable or be in-place in the plot expression
comment – a user comment (may be multiline) that will be embedded in the control file
- Returns
name of the gnuplot file created.
-
splitData
()¶ Make all plots discontinuous at this point (adds nan’s to all data fields)
-
Field¶
![digraph Field {
rankdir=LR;
margin=.2;
"Field" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Field"];
"woo.dem.DemField" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Field"];
"Field" -> "woo.dem.DemField" [arrowsize=0.5,style="setlinewidth(0.5)"]
}](_images/graphviz-669176b52b811991eff8258880799b6bb72a475c.png)
-
class
woo.core.
Field
(*args, **kwargs)¶ Spatial field described by nodes, their topology and associated values.
Overloaded function.
-
nodes
(= NodeList[])¶ Nodes referenced from this field.
[type: vector<shared_ptr<Node> >]
-
critDt
(self: woo.core.Field) → float¶ Return critical (maximum numerically stable) timestep for this field. By default returns infinity (no critical timestep) but derived fields may override this function.
-
dispHierarchy
(self: woo.core.Field, names: bool = True) → list¶ Return list of dispatch classes (from down upwards), starting with the class instance itself, top-level indexable at last. If names is true (default), return class names rather than numerical indices.
-
property
dispIndex
¶ Return class index of this instance.
-
property
nod
¶ Nodes referenced from this field.
-
property
scene
¶ Get associated scene object, if any (this function is dangerous in some corner cases, as it has to use raw pointer).
-
Node¶
-
class
woo.core.
Node
(*args, **kwargs)¶ A point in space (defining local coordinate system), referenced by other objects.
Overloaded function.
-
pos
(= Vector3(0, 0, 0))¶ Position in space (cartesian coordinates); origin \(O\) of the local coordinate system.
[type: Vector3r, unit: m]
-
ori
(= Quaternion((1, 0, 0), 0))¶ Orientation \(q\) of this node.
[type: Quaternionr]
-
data
(= [])¶ Array of data, ordered in globally consistent manner.
[type: vector<shared_ptr<NodeData> >]
-
rep
(= None)¶ What should be shown at this node when rendered via OpenGL; this data are also used in e.g. particle tracking, hence enable even in OpenGL-less builds as well.
[type: shared_ptr<
NodeVisRep
>]
-
property
dem
¶ _getDataOnNode(arg0: woo.core.Node) -> woo.dem.DemData
-
dispHierarchy
(self: woo.core.Node, names: bool = True) → list¶ Return list of dispatch classes (from down upwards), starting with the class instance itself, top-level indexable at last. If names is true (default), return class names rather than numerical indices.
-
property
dispIndex
¶ Return class index of this instance.
-
property
gl
¶ _getDataOnNode(arg0: woo.core.Node) -> woo.gl.GlData
-
glob2loc
(self: woo.core.Node, p: _wooEigen11.Vector3) → _wooEigen11.Vector3¶ Transform point \(p\) from global to node-local coordinates as \(q^*(p-O)q\), in code
q.conjugate()*(p-O)
.
-
glob2loc_rank2
(self: woo.core.Node, g: _wooEigen11.Matrix3) → _wooEigen11.Matrix3¶ Rotate rank-2 tensor (such as stress) from local to global coordinates, computed as \(\mat{R}^T\mat{g}\mat{R}\).
-
loc2glob
(self: woo.core.Node, p: _wooEigen11.Vector3) → _wooEigen11.Vector3¶ Transform point \(p_l\) from node-local to global coordinates as \(q\cdot p_l\cdot q^*+O\), in code
q*p+O
.
-
loc2glob_rank2
(self: woo.core.Node, l: _wooEigen11.Matrix3) → _wooEigen11.Matrix3¶ Rotate rank-2 tensor (such as stress), represented as 3×3 matrix, from local to global coordinates; computed as \(\mat{R}\mat{l}\mat{R}^T\), where \(\mat{R}\) is rotation matrix equivalent to rotation by quaternion
ori
.
-
property
mesh
¶ _getDataOnNode(arg0: woo.core.Node) -> woo.mesh.MeshData
-
LabelMapper¶
-
class
woo.core.
LabelMapper
(*args, **kwargs)¶ Map labels to
woo.Object
, lists ofwoo.Object
or Python’s objects, while preserving reference-counting of c++ objects during save/loads. This object is exposed asScene.labels
(with dictionary-like access) andScene.lab
(with attribute access) and presents a simulation-bound persistent namespace for arbitrary objects.Overloaded function.
__init__(self: woo.core.LabelMapper) -> None
__init__(self: woo.core.LabelMapper, *args, **kwargs) -> None
-
pyMap
(= {})¶ Map names to python objects
[type: StrPyMap, not accessible from python]
-
wooMap
(= {})¶ Map names to woo objects
[type: StrWooMap, not accessible from python]
-
wooSeqMap
(= {})¶ Map names to sequences of woo objects
[type: StrWooSeqMap, not accessible from python]
-
modSet
(= set())¶ Set of pseudo-modules names (fully qualified)
[type: std::set<string>, not accessible from python]
-
writables
(= set())¶ Set of writable names (without warning)
[type: std::set<string>, not accessible from python]
-
__contains__
(self: woo.core.LabelMapper, arg0: str) → bool¶
-
__delitem__
(self: woo.core.LabelMapper, arg0: str) → None¶
-
__dir__
(self: woo.core.LabelMapper, prefix: str = '') → list¶
-
__getitem__
(self: woo.core.LabelMapper, arg0: str) → object¶
-
__len__
(self: woo.core.LabelMapper) → int¶
-
__setitem__
(self: woo.core.LabelMapper, arg0: str, arg1: object) → None¶
-
items
(self: woo.core.LabelMapper) → list¶
-
keys
(self: woo.core.LabelMapper) → list¶
Cell¶
-
class
woo.core.
Cell
(*args, **kwargs)¶ Parameters of periodic boundary conditions. Only applies if O.isPeriodic==True.
Overloaded function.
-
trsfUpperTriangular
(= False)¶ Require that
Cell.trsf
is upper-triangular, to conform with the requirement of voro++ for sheared periodic cells.[type: bool, read-only in python]
-
trsf
(= Matrix3(1, 0, 0, 0, 1, 0, 0, 0, 1))¶ -
[type: Matrix3r]
-
refHSize
(= Matrix3(1, 0, 0, 0, 1, 0, 0, 0, 1))¶ Reference cell configuration, only used with
OpenGLRenderer.dispScale
. Updated automatically whenhSize
ortrsf
is assigned directly; also modified bywoo.utils.setRefSe3
(called e.g. by the Reference button in the UI).[type: Matrix3r]
-
hSize
(= Matrix3(1, 0, 0, 0, 1, 0, 0, 0, 1))¶ [overridden below]
[type: Matrix3r]
-
pprevHsize
(= Matrix3(1, 0, 0, 0, 1, 0, 0, 0, 1))¶ hSize at t-dt/2; used to compute velocity correction in contact with non-zero cellDist. Updated automatically.
[type: Matrix3r, read-only in python]
-
gradV
(= Matrix3(0, 0, 0, 0, 0, 0, 0, 0, 0))¶ [overridden below]
[type: Matrix3r]
-
W
(= Matrix3(0, 0, 0, 0, 0, 0, 0, 0, 0))¶ Spin tensor, computed from gradV when it is updated.
[type: Matrix3r, read-only in python]
-
spinVec
(= Vector3(0, 0, 0))¶ Angular velocity vector (1/2 * dual of spin tensor W), computed from W when it is updated.
[type: Vector3r, read-only in python]
-
nextGradV
(= Matrix3(0, 0, 0, 0, 0, 0, 0, 0, 0))¶ Value of gradV to be applied in the next step (that is, at t+dt/2). If any engine changes gradV, it should do it via this variable. The value propagates to gradV at the very end of each timestep, so if it is user-adjusted between steps, it will not become effective until after 1 steps. It should not be changed between Leapfrog and end of the step!
[type: Matrix3r]
-
homoDeform
(= 4)¶ Deform (
gradV
) the cell homothetically, by adjusting positions or velocities of particles. The values have the following meaning: 0: no homothetic deformation, 1: set absolute particle positions directly (whengradV
is non-zero), but without changing their velocity, 2: adjust particle velocity (only whengradV
changed) with Δv_i=Δ ∇v x_i. 3: as 2, but include a 2nd order term in addition – the derivative of 1 (convective term in the velocity update).[type: int, named enum, possible values are: ‘None’ (‘none’, ‘-‘; 0), ‘position only’ (‘pos’; 1), ‘pos & vel, 1st order’ (‘vel’; 2), ‘pos & vel, 2nd order’ (‘vel2’; 3), ‘leapfrog-consistent’ (‘gradV2’, ‘all’; 4)]
-
canonicalizePt
(self: woo.core.Cell, arg0: _wooEigen11.Vector3) → _wooEigen11.Vector3¶ Transform any point such that it is inside the base cell
-
property
hSize0
¶ Value of untransformed hSize, with respect to current
trsf
(computed astrsf
⁻¹ ×hSize
).
-
setBox
(*args, **kwargs)¶ Overloaded function.
setBox(self: woo.core.Cell, arg0: _wooEigen11.Vector3) -> None
Set
Cell
shape to be rectangular, with dimensions along axes specified by given argument. Shorthand for assigning diagonal matrix with respective entries tohSize
.setBox(self: woo.core.Cell, arg0: float, arg1: float, arg2: float) -> None
Set
Cell
shape to be rectangular, with dimensions along $x$, $y$, $z$ specified by arguments. Shorthand for assigning diagonal matrix with the respective entries tohSize
.
-
setCurrGradV
(self: woo.core.Cell, arg0: _wooEigen11.Matrix3) → None¶
-
shearPt
(self: woo.core.Cell, arg0: _wooEigen11.Vector3) → _wooEigen11.Vector3¶ Apply shear (cell skew+rot) on the point
-
property
shearTrsf
¶ Current skew+rot transformation (no resize)
-
property
size
¶ Current size of the cell, i.e. lengths of the 3 cell lateral vectors contained in
Cell.hSize
columns. Updated automatically at every step. Assigning a value will change the lengths of base vectors (seeCell.hSize
), keeping their orientations unchanged.
-
property
size0
¶ norms of columns of hSize0 (edge lengths of the untransformed configuration)
-
unshearPt
(self: woo.core.Cell, arg0: _wooEigen11.Vector3) → _wooEigen11.Vector3¶ Apply inverse shear on the point (removes skew+rot of the cell)
-
property
unshearTrsf
¶ Inverse of the current skew+rot transformation (no resize)
-
property
volume
¶ Current volume of the cell.
-
wrapPt
(self: woo.core.Cell, arg0: _wooEigen11.Vector3) → _wooEigen11.Vector3¶ Wrap point inside the reference cell, assuming the cell has no skew+rot.
-
EnergyTracker¶
-
class
woo.core.
EnergyTracker
(*args, **kwargs)¶ Storage for tracing energies. Only to be used if O.traceEnergy is True.
Overloaded function.
__init__(self: woo.core.EnergyTracker) -> None
__init__(self: woo.core.EnergyTracker, *args, **kwargs) -> None
-
energies
¶ Energy values, in linear array
[type: OpenMPArrayAccumulator<Real>]
-
names
(= {})¶ Associate textual name to an index in the energies array [overridden bellow].
[type: mapStringInt, not accessible from python]
-
grid
(= None)¶ Grid for tracking spatial distribution of energy increments; write-protected, use
gridOn
,gridOff
.[type: shared_ptr<
EnergyTrackerGrid
>, read-only in python]
-
flags
(= [])¶ Flags for respective energies; most importantly, whether the value should be reset at every step.
[type: vector<int>, read-only in python]
-
__contains__
(self: woo.core.EnergyTracker, arg0: str) → bool¶ Query whether given key exists; used by
'key' in EnergyTracker
-
__getitem__
(self: woo.core.EnergyTracker, arg0: str) → float¶ Get energy value for given name.
-
__iter__
(self: woo.core.EnergyTracker) → EnergyTracker::pyIterator¶ Return iterator over keys, to support python iteration protocol.
-
__len__
(self: woo.core.EnergyTracker) → int¶ Number of items in the container.
-
__setitem__
(self: woo.core.EnergyTracker, arg0: str, arg1: float) → None¶ Set energy value for given name (will create a non-resettable item, if it does not exist yet).
-
add
(self: woo.core.EnergyTracker, dE: float, name: str, reset: bool = False) → None¶ Accumulate energy, used from python (likely inefficient)
-
clear
(self: woo.core.EnergyTracker) → None¶ Clear all stored values.
-
gridOff
(self: woo.core.EnergyTracker) → None¶ Disable
grid
so that energy location is not recorded anymore. Thegrid
object is discarded, including any data it might have contained.
-
gridOn
(self: woo.core.EnergyTracker, box: _wooEigen11.AlignedBox3, cellSize: float, maxIndex: int = - 1) → None¶ Initialize
grid
object, which will record spacial location of energy events.
-
gridToVTK
(self: woo.core.EnergyTracker, out: str) → str¶ Write grid data to VTK file out (
.vti
will be appended); returns output file name.
-
items
(self: woo.core.EnergyTracker) → list¶ Return contents as list of (name,value) tuples.
-
keys
(self: woo.core.EnergyTracker) → list¶ Return defined energies.
-
relErr
(self: woo.core.EnergyTracker) → float¶ Total energy divided by sum of absolute values.
-
total
(self: woo.core.EnergyTracker) → float¶ Return sum of all energies.
Engine¶
![digraph Engine {
rankdir=LR;
margin=.2;
"Engine" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Engine"];
"woo.gl.GlFieldDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Engine"];
"Dispatcher" -> "woo.gl.GlFieldDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.VtkExport" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.VtkExport" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.PeriIsoCompressor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.PeriIsoCompressor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.GridBoundDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Dispatcher" -> "woo.dem.GridBoundDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlShapeDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Engine"];
"Dispatcher" -> "woo.gl.GlShapeDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.AnisoPorosityAnalyzer" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.AnisoPorosityAnalyzer" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.GridCollider" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.Collider" -> "woo.dem.GridCollider" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.LawDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Dispatcher" -> "woo.dem.LawDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.DynDt" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.DynDt" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.FlowAnalysis" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.FlowAnalysis" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoxOutlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.Outlet" -> "woo.dem.BoxOutlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ArcOutlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.Outlet" -> "woo.dem.ArcOutlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Tracer" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.Tracer" [arrowsize=0.5,style="setlinewidth(0.5)"] "PyRunner" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Engine"];
"PeriodicEngine" -> "PyRunner" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.POVRayExport" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.POVRayExport" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.WeirdTriaxControl" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.WeirdTriaxControl" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.PelletAgglomerator" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.PelletAgglomerator" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ForceResetter" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.ForceResetter" [arrowsize=0.5,style="setlinewidth(0.5)"] "WooTestPeriodicEngine" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Engine"];
"PeriodicEngine" -> "WooTestPeriodicEngine" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Outlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.Outlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.DetectSteadyState" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.DetectSteadyState" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.AabbCollider" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.Collider" -> "woo.dem.AabbCollider" [arrowsize=0.5,style="setlinewidth(0.5)"] "ParallelEngine" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Engine"];
"Engine" -> "ParallelEngine" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.HalfspaceBuoyancy" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.HalfspaceBuoyancy" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.CPhysDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Dispatcher" -> "woo.dem.CPhysDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.StackedBoxOutlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.BoxOutlet" -> "woo.dem.StackedBoxOutlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Suspicious" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.Suspicious" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ForcesToHdf5" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.ForcesToHdf5" [arrowsize=0.5,style="setlinewidth(0.5)"] "PeriodicEngine" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Engine"];
"Engine" -> "PeriodicEngine" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.MeshVolume" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.MeshVolume" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Inlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.Inlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.AabbTreeCollider" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.AabbCollider" -> "woo.dem.AabbTreeCollider" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Leapfrog" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.Leapfrog" [arrowsize=0.5,style="setlinewidth(0.5)"] "Dispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Engine"];
"Engine" -> "Dispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.InsertionSortCollider" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.AabbCollider" -> "woo.dem.InsertionSortCollider" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoundDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Dispatcher" -> "woo.dem.BoundDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.CylinderInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.RandomInlet" -> "woo.dem.CylinderInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ArcInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.RandomInlet" -> "woo.dem.ArcInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlNodeDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Engine"];
"Dispatcher" -> "woo.gl.GlNodeDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ClusterAnalysis" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.ClusterAnalysis" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ContactLoop" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.ContactLoop" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlCPhysDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Engine"];
"Dispatcher" -> "woo.gl.GlCPhysDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo._qt.SnapshotEngine" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo._qt.html#woo._qt.Engine"];
"PeriodicEngine" -> "woo._qt.SnapshotEngine" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.CGeomDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Dispatcher" -> "woo.dem.CGeomDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.IntraForce" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Dispatcher" -> "woo.dem.IntraForce" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoxInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.RandomInlet" -> "woo.dem.BoxInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.RandomInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.Inlet" -> "woo.dem.RandomInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Collider" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.Collider" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoxTraceTimeSetter" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"PeriodicEngine" -> "woo.dem.BoxTraceTimeSetter" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoxInlet2d" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.BoxInlet" -> "woo.dem.BoxInlet2d" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlBoundDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Engine"];
"Dispatcher" -> "woo.gl.GlBoundDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ConveyorInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.Inlet" -> "woo.dem.ConveyorInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.AxialGravity" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.AxialGravity" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.NodalForcesToHdf5" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"woo.dem.ForcesToHdf5" -> "woo.dem.NodalForcesToHdf5" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.LawTester" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Engine"];
"Engine" -> "woo.dem.LawTester" [arrowsize=0.5,style="setlinewidth(0.5)"]
}](_images/graphviz-ff6c09880a0750110d4f60898f7167ef85b27084.png)
-
class
woo.core.
Engine
(*args, **kwargs)¶ Basic execution unit of simulation, called from the simulation loop (O.engines)
Overloaded function.
-
dead
(= False)¶ If true, this engine will not run at all; can be used for making an engine temporarily deactivated and only resurrect it at a later point.
[type: bool]
-
label
(= '')¶ Textual label for this object; must be valid python identifier, you can refer to it directly from python.
[type: string, not shown in the UI]
-
field
(= None)¶ User-requested woo.core.Field to run this engine on; if empty, fields will be searched for admissible ones; if more than one is found, exception will be raised.
[type: shared_ptr<
Field
>, not shown in the UI, not dumped]
-
userAssignedField
(= False)¶ Whether the woo.core.Engine.field was user-assigned or automatically assigned, to know whether to update automatically.
[type: bool, not shown in the UI, read-only in python]
-
isNewObject
(= True)¶ Flag to recognize in postLoad whether this object has just been constructed, to set userAssignedField properly (ugly…)
[type: bool, not accessible from python]
-
__call__
(self: woo.core.Engine, scene: woo.core.Scene, field: woo.core.Field = None) → None¶ Run the engine just once, using scene. If field is not given as the engine requires it, it will be obtained from scene automatically (with the same rules as for engines which don’t have an explicit field: if one field is found, it is used, no or more compatible fields raise an exception.)
-
acceptsField
(self: woo.core.Engine, arg0: woo.core.Field) → bool¶
-
critDt
(self: woo.core.Engine) → float¶ Return critical (maximum numerically stable) timestep for this engine. By default returns infinity (no critical timestep) but derived engines may override this function.
-
property
execCount
¶ Cummulative count this engine was run (only used if
Master.timingEnabled
==True
).
-
property
execTime
¶ Cummulative time this Engine took to run (only used if
Master.timingEnabled
==True
).
-
property
scene
¶ Get associated scene object, if any (this function is dangerous in some corner cases, as it has to use raw pointer).
-
property
timingDeltas
¶ Detailed information about timing inside the Engine itself. Empty unless enabled in the source code and
Master.timingEnabled
==True
.
-
ParallelEngine¶
Object
→ Engine
→ ParallelEngine
-
class
woo.core.
ParallelEngine
(*args, **kwargs)¶ Engine for running other Engine in parallel.
Special constructor
Possibly nested list of engines, where each top-level item (engine or list) will be run in parallel; nested lists will be run sequentially.
Overloaded function.
__init__(self: woo.core.ParallelEngine) -> None
__init__(self: woo.core.ParallelEngine, *args, **kwargs) -> None
-
slaves
(= [])¶ [will be overridden]
[type: slaveContainer, not accessible from python]
Dispatcher¶
Object
→ Engine
→ Dispatcher
![digraph Dispatcher {
rankdir=LR;
margin=.2;
"Dispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Dispatcher"];
"woo.gl.GlFieldDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Dispatcher"];
"Dispatcher" -> "woo.gl.GlFieldDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.GridBoundDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Dispatcher"];
"Dispatcher" -> "woo.dem.GridBoundDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlShapeDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Dispatcher"];
"Dispatcher" -> "woo.gl.GlShapeDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.CPhysDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Dispatcher"];
"Dispatcher" -> "woo.dem.CPhysDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoundDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Dispatcher"];
"Dispatcher" -> "woo.dem.BoundDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlBoundDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Dispatcher"];
"Dispatcher" -> "woo.gl.GlBoundDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.LawDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Dispatcher"];
"Dispatcher" -> "woo.dem.LawDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlNodeDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Dispatcher"];
"Dispatcher" -> "woo.gl.GlNodeDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlCPhysDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Dispatcher"];
"Dispatcher" -> "woo.gl.GlCPhysDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.CGeomDispatcher" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Dispatcher"];
"Dispatcher" -> "woo.dem.CGeomDispatcher" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.IntraForce" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Dispatcher"];
"Dispatcher" -> "woo.dem.IntraForce" [arrowsize=0.5,style="setlinewidth(0.5)"]
}](_images/graphviz-bad7e6c3953371b9249d3ed3b8fa53c60564a577.png)
-
class
woo.core.
Dispatcher
(*args, **kwargs)¶ Engine dispatching control to its associated functors, based on types of argument it receives. This abstract base class provides no functionality in itself.
Overloaded function.
Functor¶
![digraph Functor {
rankdir=LR;
margin=.2;
"Functor" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.Functor"];
"woo.dem.In2_Facet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.IntraFunctor" -> "woo.dem.In2_Facet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Ellipsoid_Ellipsoid_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Ellipsoid_Ellipsoid_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoundFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"Functor" -> "woo.dem.BoundFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlBoundFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"Functor" -> "woo.gl.GlBoundFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Tetra" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlShapeFunctor" -> "woo.gl.Gl1_Tetra" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Rod" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlShapeFunctor" -> "woo.gl.Gl1_Rod" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cp2_ConcreteMat_ConcretePhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.CPhysFunctor" -> "woo.dem.Cp2_ConcreteMat_ConcretePhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.In2_Truss_ElastMat" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.IntraFunctor" -> "woo.dem.In2_Truss_ElastMat" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Capsule" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.Gl1_Sphere" -> "woo.gl.Gl1_Capsule" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Wall_Sphere_G3Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.CGeomFunctor" -> "woo.dem.Cg2_Wall_Sphere_G3Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Wall_Ellipsoid_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Wall_Ellipsoid_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Wall" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlShapeFunctor" -> "woo.gl.Gl1_Wall" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Grid1_Facet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.GridBoundFunctor" -> "woo.dem.Grid1_Facet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_DemField" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlFieldFunctor" -> "woo.gl.Gl1_DemField" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cp2_PelletMat_PelletPhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cp2_FrictMat_FrictPhys" -> "woo.dem.Cp2_PelletMat_PelletPhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.CPhysFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"Functor" -> "woo.dem.CPhysFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Facet_Facet_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Facet_Facet_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Bo1_Facet_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.BoundFunctor" -> "woo.dem.Bo1_Facet_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Law2_L6Geom_FrictPhys_LinEl6" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.LawFunctor" -> "woo.dem.Law2_L6Geom_FrictPhys_LinEl6" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.fem.In2_Membrane_FrictMat" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.fem.html#woo.fem.Functor"];
"woo.fem.In2_Membrane_ElastMat" -> "woo.fem.In2_Membrane_FrictMat" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Grid1_Wall" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.GridBoundFunctor" -> "woo.dem.Grid1_Wall" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Bo1_Wall_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.BoundFunctor" -> "woo.dem.Bo1_Wall_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.In2_Sphere_ElastMat" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.IntraFunctor" -> "woo.dem.In2_Sphere_ElastMat" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Sphere_Sphere_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Sphere_Sphere_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_InfCylinder" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlShapeFunctor" -> "woo.gl.Gl1_InfCylinder" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.GridBoundFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"Functor" -> "woo.dem.GridBoundFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Bo1_Cone_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.BoundFunctor" -> "woo.dem.Bo1_Cone_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Wall_Sphere_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Wall_Sphere_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Any_Any_L6Geom__Base" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.CGeomFunctor" -> "woo.dem.Cg2_Any_Any_L6Geom__Base" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_InfCylinder_Capsule_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_InfCylinder_Capsule_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlFieldFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"Functor" -> "woo.gl.GlFieldFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Law2_L6Geom_LudingPhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.LawFunctor" -> "woo.dem.Law2_L6Geom_LudingPhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Law2_L6Geom_FrictPhys_IdealElPl" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.LawFunctor" -> "woo.dem.Law2_L6Geom_FrictPhys_IdealElPl" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Tet4" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.Gl1_Tetra" -> "woo.gl.Gl1_Tet4" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Facet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlShapeFunctor" -> "woo.gl.Gl1_Facet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Wall_Capsule_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Wall_Capsule_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_InfCylinder_Sphere_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_InfCylinder_Sphere_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Sphere_Ellipsoid_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Ellipsoid_Ellipsoid_L6Geom" -> "woo.dem.Cg2_Sphere_Ellipsoid_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.IntraFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"Functor" -> "woo.dem.IntraFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.fem.Bo1_Tetra_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.fem.html#woo.fem.Functor"];
"woo.dem.BoundFunctor" -> "woo.fem.Bo1_Tetra_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlCPhysFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"Functor" -> "woo.gl.GlCPhysFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Cone_Sphere_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Cone_Sphere_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Law2_L6Geom_ConcretePhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.LawFunctor" -> "woo.dem.Law2_L6Geom_ConcretePhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Bo1_Capsule_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.BoundFunctor" -> "woo.dem.Bo1_Capsule_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cp2_IceMat_IcePhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cp2_FrictMat_FrictPhys" -> "woo.dem.Cp2_IceMat_IcePhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlShapeFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"Functor" -> "woo.gl.GlShapeFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_ConcretePhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.Gl1_CPhys" -> "woo.gl.Gl1_ConcretePhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Facet_Ellipsoid_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Facet_Ellipsoid_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Law2_L6Geom_PelletPhys_Pellet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.LawFunctor" -> "woo.dem.Law2_L6Geom_PelletPhys_Pellet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Law2_G3Geom_FrictPhys_IdealElPl" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.LawFunctor" -> "woo.dem.Law2_G3Geom_FrictPhys_IdealElPl" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_GridBound" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlBoundFunctor" -> "woo.gl.Gl1_GridBound" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Facet_InfCylinder_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Facet_InfCylinder_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.CGeomFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"Functor" -> "woo.dem.CGeomFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.LawFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"Functor" -> "woo.dem.LawFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Bo1_Rod_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.BoundFunctor" -> "woo.dem.Bo1_Rod_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cp2_HertzMat_HertzPhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cp2_FrictMat_FrictPhys" -> "woo.dem.Cp2_HertzMat_HertzPhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlBoundFunctor" -> "woo.gl.Gl1_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cp2_FrictMat_FrictPhys_CrossAnisotropic" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.CPhysFunctor" -> "woo.dem.Cp2_FrictMat_FrictPhys_CrossAnisotropic" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Sphere_Sphere_G3Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.CGeomFunctor" -> "woo.dem.Cg2_Sphere_Sphere_G3Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Bo1_Ellipsoid_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Bo1_Sphere_Aabb" -> "woo.dem.Bo1_Ellipsoid_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Grid1_InfCylinder" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.GridBoundFunctor" -> "woo.dem.Grid1_InfCylinder" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Membrane" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.Gl1_Facet" -> "woo.gl.Gl1_Membrane" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Rod_Sphere_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Rod_Sphere_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Sphere" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlShapeFunctor" -> "woo.gl.Gl1_Sphere" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Capsule_Capsule_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Capsule_Capsule_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Node" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlNodeFunctor" -> "woo.gl.Gl1_Node" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.fem.In2_Tet4_ElastMat" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.fem.html#woo.fem.Functor"];
"woo.dem.IntraFunctor" -> "woo.fem.In2_Tet4_ElastMat" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.GlNodeFunctor" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"Functor" -> "woo.gl.GlNodeFunctor" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.fem.In2_Membrane_ElastMat" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.fem.html#woo.fem.Functor"];
"woo.dem.In2_Facet" -> "woo.fem.In2_Membrane_ElastMat" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Facet_Sphere_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Facet_Sphere_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Wall_Facet_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Wall_Facet_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cp2_FrictMat_FrictPhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.CPhysFunctor" -> "woo.dem.Cp2_FrictMat_FrictPhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Bo1_Sphere_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.BoundFunctor" -> "woo.dem.Bo1_Sphere_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Grid1_Sphere" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.GridBoundFunctor" -> "woo.dem.Grid1_Sphere" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_CPhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlCPhysFunctor" -> "woo.gl.Gl1_CPhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.In2_Wall_ElastMat" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.IntraFunctor" -> "woo.dem.In2_Wall_ElastMat" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Ellipsoid" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.Gl1_Sphere" -> "woo.gl.Gl1_Ellipsoid" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.Gl1_Cone" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.Functor"];
"woo.gl.GlShapeFunctor" -> "woo.gl.Gl1_Cone" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Law2_L6Geom_IcePhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.LawFunctor" -> "woo.dem.Law2_L6Geom_IcePhys" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Bo1_InfCylinder_Aabb" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.BoundFunctor" -> "woo.dem.Bo1_InfCylinder_Aabb" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Law2_L6Geom_HertzPhys_DMT" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.LawFunctor" -> "woo.dem.Law2_L6Geom_HertzPhys_DMT" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Sphere_Capsule_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Sphere_Capsule_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cg2_Facet_Capsule_L6Geom" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cg2_Any_Any_L6Geom__Base" -> "woo.dem.Cg2_Facet_Capsule_L6Geom" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Cp2_LudingMat_LudingPhys" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.Functor"];
"woo.dem.Cp2_FrictMat_FrictPhys" -> "woo.dem.Cp2_LudingMat_LudingPhys" [arrowsize=0.5,style="setlinewidth(0.5)"]
}](_images/graphviz-c6bcdf7883e28716d1b61a45b66d220522a6d5d7.png)
-
class
woo.core.
Functor
(*args, **kwargs)¶ Function-like object that is called by Dispatcher, if types of arguments match those the Functor declares to accept.
Overloaded function.
-
label
(= '')¶ Textual label for this object; must be valid python identifier, you can refer to it directly fron python (must be a valid python identifier).
[type: string, not shown in the UI]
-
property
bases
¶ Ordered list of types (as strings) this functor accepts.
-
property
timingDeltas
¶ Detailed information about timing inside the Dispatcher itself. Empty unless enabled in the source code and
Master.timingEnabled
isTrue
.
-
PeriodicEngine¶
Object
→ Engine
→ PeriodicEngine
![digraph PeriodicEngine {
rankdir=LR;
margin=.2;
"PeriodicEngine" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.PeriodicEngine"];
"woo.dem.Inlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.Inlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.VtkExport" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.VtkExport" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.CylinderInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.RandomInlet" -> "woo.dem.CylinderInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ArcInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.RandomInlet" -> "woo.dem.ArcInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.DynDt" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.DynDt" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.FlowAnalysis" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.FlowAnalysis" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoxOutlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.Outlet" -> "woo.dem.BoxOutlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ClusterAnalysis" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.ClusterAnalysis" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ArcOutlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.Outlet" -> "woo.dem.ArcOutlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Tracer" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.Tracer" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo._qt.SnapshotEngine" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo._qt.html#woo._qt.PeriodicEngine"];
"PeriodicEngine" -> "woo._qt.SnapshotEngine" [arrowsize=0.5,style="setlinewidth(0.5)"] "PyRunner" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.PeriodicEngine"];
"PeriodicEngine" -> "PyRunner" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoxInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.RandomInlet" -> "woo.dem.BoxInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.RandomInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.Inlet" -> "woo.dem.RandomInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.POVRayExport" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.POVRayExport" [arrowsize=0.5,style="setlinewidth(0.5)"] "WooTestPeriodicEngine" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.PeriodicEngine"];
"PeriodicEngine" -> "WooTestPeriodicEngine" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Outlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.Outlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.DetectSteadyState" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.DetectSteadyState" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoxTraceTimeSetter" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.BoxTraceTimeSetter" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.BoxInlet2d" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.BoxInlet" -> "woo.dem.BoxInlet2d" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ConveyorInlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.Inlet" -> "woo.dem.ConveyorInlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.StackedBoxOutlet" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.BoxOutlet" -> "woo.dem.StackedBoxOutlet" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.Suspicious" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.Suspicious" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.ForcesToHdf5" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.ForcesToHdf5" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.NodalForcesToHdf5" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"woo.dem.ForcesToHdf5" -> "woo.dem.NodalForcesToHdf5" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.MeshVolume" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.PeriodicEngine"];
"PeriodicEngine" -> "woo.dem.MeshVolume" [arrowsize=0.5,style="setlinewidth(0.5)"]
}](_images/graphviz-ed6304d2bcfd5de05e5488ba39aa3f51c4c5a5ff.png)
-
class
woo.core.
PeriodicEngine
(*args, **kwargs)¶ Run Engine::run with given fixed periodicity real time (=wall clock time, computation time), virtual time (simulation time), step number), by setting any of those criteria (virtPeriod, realPeriod, stepPeriod) to a positive value. They are all negative (inactive) by default.
The number of times this engine is activated can be limited by setting nDo>0. If the number of activations will have been already reached, no action will be called even if an active period has elapsed.
If initRun is set (true by default), the engine will run when called for the first time; otherwise it will only start counting period (realLast etc interal variables) from that point, but without actually running, and will run only once a period has elapsed since the initial run.
This class should not be used directly; rather, derive your own engine which you want to be run periodically.
Derived engines should override Engine::action(), which will be called periodically. If the derived Engine overrides also Engine::isActivated, it should also take in account return value from PeriodicEngine::isActivated, otherwise the periodicity will not be functional.
Example with PyRnner, which derives from PeriodicEngine; likely to be encountered in python scripts):
PyRunner(realPeriod=5,stepPeriod=10000,command='print O.step') will print step number every 10000 step or every 5 seconds of wall clock time, whiever comes first since it was last run.
Overloaded function.
__init__(self: woo.core.PeriodicEngine) -> None
__init__(self: woo.core.PeriodicEngine, *args, **kwargs) -> None
-
virtPeriod
(= 0.0)¶ Periodicity criterion using virtual (simulation) time (deactivated if <= 0)
[type: Real]
-
realPeriod
(= 0.0)¶ Periodicity criterion using real (wall clock, computation, human) time (deactivated if <=0)
[type: Real]
-
stepPeriod
(= 1)¶ Periodicity criterion using step number (deactivated if <= 0)
[type: long]
-
stepModulo
(= True)¶ If true, interpret
stepPeriod
as modulo value forScene.step
. This is useful to assure two engines will run within the same step regardless of when they were started. This may lead to Engine being run earlier thanstepPeriod
after the last run, in casestepPeriod
is changed.[type: bool]
-
nDo
(= -1)¶ Limit number of executions by this number (deactivated if negative)
[type: long]
-
nDone
(= 0)¶ Track number of executions (cumulative).
[type: long]
-
initRun
(= True)¶ Run the first time we are called as well.
[type: bool]
-
virtLast
(= nan)¶ Tracks virtual time of last run.
[type: Real]
-
realLast
(= nan)¶ Tracks real time of last run.
[type: Real]
-
stepLast
(= -1)¶ Tracks step number of last run.
[type: long]
-
stepPrev
(= -1)¶ Number of step when we run previously (stepLast is the current step when the engine runs)
[type: long, not shown in the UI]
-
virtPrev
(= -1.0)¶ Simulation time when run previously
[type: Real, not shown in the UI]
-
realPrev
(= -1.0)¶ Real time when run previously
[type: Real, not shown in the UI]
WooTestPeriodicEngine¶
Object
→ Engine
→ PeriodicEngine
→ WooTestPeriodicEngine
-
class
woo.core.
WooTestPeriodicEngine
(*args, **kwargs)¶ Test some PeriodicEngine features.
Overloaded function.
__init__(self: woo.core.WooTestPeriodicEngine) -> None
__init__(self: woo.core.WooTestPeriodicEngine, *args, **kwargs) -> None
-
deadCounter
(= 0)¶ Count how many times
dead
was assigned to.[type: int]
PyRunner¶
Object
→ Engine
→ PeriodicEngine
→ PyRunner
-
class
woo.core.
PyRunner
(*args, **kwargs)¶ Execute a python command periodically, with defined (and adjustable) periodicity. See
PeriodicEngine
documentation for details.Special constructor
command can be given as first unnamed string argument (
PyRunner('foo()')
), stepPeriod as unnamed integer argument (PyRunner('foo()',100)
orPyRunner(100,'foo()')
).Overloaded function.
__init__(self: woo.core.PyRunner) -> None
-
command
(= '')¶ Command to be run by python interpreter. Not run if empty.
[type: string]
WooTestClass¶
-
class
woo.core.
WooTestClass
(*args, **kwargs)¶ This class serves to test various functionalities; it also includes all possible units, which thus get registered in woo
Overloaded function.
__init__(self: woo.core.WooTestClass) -> None
__init__(self: woo.core.WooTestClass, *args, **kwargs) -> None
-
angle
(= 0.0)¶ Variable with angle unit.
[type: Real, unit: rad]
-
time
(= 0.0)¶ Variable with time unit.
[type: Real, unit: s]
-
len
(= 0.0)¶ Variable with len unit.
[type: Real, unit: m]
-
length_with_inches
(= 0.0)¶ Variable with length, but also showing inches in the UI
[type: Real, unit: m]
-
area
(= 0.0)¶ Variable with area unit.
[type: Real, unit: m²]
-
vol
(= 0.0)¶ Variable with vol unit.
[type: Real, unit: m³]
-
vel
(= 0.0)¶ Variable with vel unit.
[type: Real, unit: m/s]
-
accel
(= 0.0)¶ Variable with accel unit.
[type: Real, unit: m/s²]
-
mass
(= 0.0)¶ Variable with mass unit.
[type: Real, unit: kg]
-
angVel
(= 0.0)¶ Variable with angVel unit.
[type: Real, unit: rad/s]
-
angMom
(= 0.0)¶ Variable with angMom unit.
[type: Real, unit: N·m·s]
-
inertia
(= 0.0)¶ Variable with inertia unit.
[type: Real, unit: kg·m²]
-
force
(= 0.0)¶ Variable with force unit.
[type: Real, unit: N]
-
torque
(= 0.0)¶ Variable with torque unit.
[type: Real, unit: N·m]
-
pressure
(= 0.0)¶ Variable with pressure unit.
[type: Real, unit: Pa]
-
stiffness
(= 0.0)¶ Variable with stiffness unit.
[type: Real, unit: Pa]
-
massRate
(= 0.0)¶ Variable with massRate unit.
[type: Real, unit: kg/s]
-
density
(= 0.0)¶ Variable with density unit.
[type: Real, unit: kg/m³]
-
fraction
(= 0.0)¶ Variable with fraction unit.
[type: Real, unit: -]
-
surfEnergy
(= 0.0)¶ Variable with surfEnergy unit.
[type: Real, unit: J/m²]
-
aaccu
¶ Test openmp array accumulator
[type: OpenMPArrayAccumulator<Real>, not shown in the UI, not dumped]
-
noSaveAttr
(= 0)¶ Attribute which is not saved
[type: int, not saved]
Hidden data member (not accessible from python)
[type: int, not accessible from python]
-
noDumpAttr
(= 0)¶ Attribute with dumping disabled (trait template parameter)
[type: int, not dumped]
-
noDumpAttr2
(= 0)¶ Attribute with dumping disabled (trait method modifier)
[type: int, not dumped]
-
noDumpMaybe
(= 0)¶ Attribute with dumping disabled (but saving enabled) depending on the value of
noDumpCondition
.[type: int]
-
noDumpCondition
(= True)¶ Attribute influencing whether
noDumpMaybe
will be dumped (when false) or not (when true)[type: bool]
-
meaning42
(= 42)¶ Read-only data member
[type: int, read-only in python]
-
foo_incBaz
(= 0)¶ Change this attribute to have baz incremented
[type: int]
-
bar_zeroBaz
(= 0)¶ Change this attribute to have baz incremented
[type: int]
-
baz
(= 0)¶ Value which is changed when assigning to foo_incBaz / bar_zeroBaz.
[type: int]
-
postLoadStage
(= -1)¶ Store the last stage from postLoad (to check it is called the right way)
[type: int, read-only in python]
-
matX
(= MatrixX())¶ MatriXr object, for testing serialization of arrays.
[type: MatrixXr]
-
arr3d
¶ boost::multi_array<Real,3> object for testing serialization of multi_array.
[type: boost_multi_array_real_3, not accessible from python]
-
namedEnum
(= -1)¶ Named enumeration.
[type: int, named enum, possible values are: ‘minus one’ (‘_1’, ‘neg1’; -1), ‘zero’ (‘nothing’, ‘NULL’; 0), ‘one’ (‘single’; 1), ‘two’ (‘double’, ‘2’; 2)]
-
bits
(= 0)¶ Test writable bits of writable flags var.
[type: int, bit accessors: bit0, bit1, bit2, bit3, bit4]
-
bitsRw
(= 0)¶ Test writable bits of read-only flags var.
[type: int, read-only in python, bit accessors: bit0rw, bit1rw, bit2rw, bit3rw, bit4rw]
-
bitsRo
(= 3)¶ Test read-only bits of read-only flags var.
[type: int, read-only in python, bit accessors: bit0ro, bit1ro, bit2ro, bit3ro, bit4ro]
-
strVar
(= '')¶ Test string type var.
[type: string]
-
deprecatedAttr
(= -1)¶ deprecated, and this exaplins why…
[type: int, not shown in the UI, not dumped, DEPRECATED, raises
ValueError
when accessed]
-
objList
(= ObjectList[0x15da440, 0x15da470])¶ Testing opaque object list.
[type: vector<shared_ptr<Object>>, read-only in python]
-
property
aaccuRaw
¶ Access OpenMPArrayAccumulator data directly. Writing resizes and sets the 0th thread value, resetting all other ones.
-
aaccuWriteThreads
(self: woo.core.WooTestClass, index: int, cycleData: List[float]) → None¶ Assign a single line in the array accumulator, assigning number from cycleData in parallel in each thread.
-
arr3d_set
(self: woo.core.WooTestClass, shape: _wooEigen11.Vector3i, data: List[float]) → None¶ Set arr3d to have shape and fill it with data (must have the corresponding number of elements).
NodeVisRep¶
![digraph NodeVisRep {
rankdir=LR;
margin=.2;
"NodeVisRep" [shape="box",fontsize=8,style="setlinewidth(0.5),solid",height=0.2,URL="woo.core.html#woo.core.NodeVisRep"];
"woo.gl.LabelGlRep" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.NodeVisRep"];
"NodeVisRep" -> "woo.gl.LabelGlRep" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.ActReactGlRep" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.NodeVisRep"];
"woo.gl.VectorGlRep" -> "woo.gl.ActReactGlRep" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.TensorGlRep" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.NodeVisRep"];
"NodeVisRep" -> "woo.gl.TensorGlRep" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.dem.TraceVisRep" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.dem.html#woo.dem.NodeVisRep"];
"NodeVisRep" -> "woo.dem.TraceVisRep" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.CylGlRep" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.NodeVisRep"];
"NodeVisRep" -> "woo.gl.CylGlRep" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.ScalarGlRep" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.NodeVisRep"];
"NodeVisRep" -> "woo.gl.ScalarGlRep" [arrowsize=0.5,style="setlinewidth(0.5)"] "woo.gl.VectorGlRep" [shape="box",fontsize=8,style="setlinewidth(0.5),filled",fillcolor=grey,height=0.2,URL="woo.gl.html#woo.gl.NodeVisRep"];
"NodeVisRep" -> "woo.gl.VectorGlRep" [arrowsize=0.5,style="setlinewidth(0.5)"]
}](_images/graphviz-5f490c5fc50d8933809c068e3a45442c46bae0f8.png)
MatchMaker¶
-
class
woo.core.
MatchMaker
(*args, **kwargs)¶ Class matching pair of ids to return pre-defined (for a pair of ids defined in
matches
) or derived value (computed usingalgo
) of a scalar parameter. It can be called (id1
,id2
,val1=NaN
,val2=NaN
) in both python and c++.Note
There is a
converter
from python number defined for this class, which creates a newMatchMaker
returning the value of that number; instead of giving the object instance therefore, you can only pass the number value and it will be converted automatically.Overloaded function.
__init__(self: woo.core.MatchMaker) -> None
__init__(self: woo.core.MatchMaker, *args, **kwargs) -> None
-
matches
(= [])¶ Array of
(id1,id2,value)
items; queries matchingid1
+id2
orid2
+id1
will returnvalue
[type: std::vector<Vector3r>]
-
algo
(= 'avg')¶ Alogorithm used to compute value when no match for ids is found. Possible values are
‘avg’ (arithmetic average)
‘min’ (minimum value)
‘max’ (maximum value)
‘harmAvg’ (harmonic average)
The following algo algorithms do not require meaningful input values in order to work:
‘val’ (return value specified by
val
)‘zero’ (always return 0.)
[type: std::string]
-
__call__
(self: woo.core.MatchMaker, id1: int, id2: int, val1: float = nan, val2: float = nan) → float¶ Ask the instance for scalar value for given pair id1,*id2* (the order is irrelevant). Optionally, val1, val2 can be given so that if there is no
match
, return value can be computed using givenalgo
. If there is no match and val1, val2 are not given, an exception is raised.
-
class
woo.core.
Object
(self: woo.core.Object) → None¶ Base class for all Woo classes, providing uniform interface for constructors with attributes, attribute access, pickling, serialization via cereal/boost::serialization, equality comparison, attribute traits.
-
__eq__
(self: woo.core.Object, arg0: woo.core.Object) → bool¶
-
__ne__
(self: woo.core.Object, arg0: woo.core.Object) → bool¶
-
__repr__
(self: woo.core.Object) → str¶
-
__str__
(self: woo.core.Object) → str¶
-
deepcopy
(**kw)¶ Make object deepcopy by serializing to memory and deserializing.
-
dict
(self: woo.core.Object, all: bool = True) → dict¶ Return dictionary of attributes; all will cause also attributed with the
noSave
ornoDump
flags to be returned.
-
dump
(out, format='auto', fallbackFormat=None, overwrite=True, fragment=False, width=80, noMagic=False, showDoc=False, hideWooExtra=False)¶ Dump an object in specified format; out can be a str (filename) or a file object. Supported formats are: auto (auto-detected from out extension; raises exception when out is an object), html, expr.
-
classmethod
loads
(data, format='auto', overrideHashPercent={})¶ Load object from file, with format auto-detection; when typ is None, no type-checking is performed.
-
save
(self: woo.core.Object, filename: str) → None¶
-
updateAttrs
(self: woo.core.Object, arg0: dict) → None¶ Update object attributes from given dictionary
-
Tip
Report issues or inclarities to github.